Core PHP Programming, Third Edition is the authoritative guide to the new PHP 5 for experienced developers. Top PHP developer Leon Atkinson and PHP 5 contributor/Zend Engine 2 co-creator Zeev Suraski cover every facet of real-world PHP 5 development, from basic syntax to advanced object--oriented development-even design patterns!
It's all here: networking, data structures, regular expressions, math, configuration, graphics, MySQL/PostgreSQL support, XML, algorithms, debugging, optimization...and 650 downloadable code examples, with a Foreword by PHP 5 contributor and Zend Engine 2 co-creator Andi Gutmans!
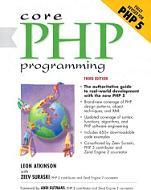
Copyright
Praise for Core PHP Programming
Prentice Hall PTR Core Series
About Prentice Hall Professional Technical Reference
Foreword
Preface
Acknowledgments
Part I. Programming with PHP
Chapter 1. An Introduction to PHP
Section 1.1. The Origins of PHP
Section 1.2. PHP Is Better Than Its Alternatives
Section 1.3. Interfaces to External Systems
Section 1.4. How PHP Works with the Web Server
Section 1.5. Hardware and Software Requirements
Section 1.6. What a PHP Script Looks Like
Section 1.7. Saving Data for Later
Section 1.8. Receiving User Input
Section 1.9. Choosing Between Alternatives
Section 1.10. Repeating Code
Chapter 2. Variables, Operators, and Expressions
Section 2.1. A Top-Down View
Section 2.2. Data Types
Section 2.3. Variables
Section 2.4. Constants
Section 2.5. Operators
Section 2.6. Building Expressions
Chapter 3. Control Statements
Section 3.1. The if Statement
Section 3.2. The ? Operator
Section 3.3. The switch Statement
Section 3.4. Loops
Section 3.5. exit, die, and return
Section 3.6. Exceptions
Section 3.7. Declare
Chapter 4. Functions
Section 4.1. Declaring a Function
Section 4.2. The return Statement
Section 4.3. Scope
Section 4.4. Static Variables
Section 4.5. Arguments
Section 4.6. Recursion
Section 4.7. Dynamic Function Calls
Chapter 5. Arrays
Section 5.1. Single-Dimensional Arrays
Section 5.2. Indexing Arrays
Section 5.3. Initializing Arrays
Section 5.4. Multidimensional Arrays
Section 5.5. Casting Arrays
Section 5.6. The + Operator
Section 5.7. Referencing Arrays Inside Strings
Chapter 6. Classes and Objects
Section 6.1. Object-Oriented Programming
Section 6.2. The PHP 5 Object Model
Section 6.3. Defining a Class
Section 6.4. Constructors and Destructors
Section 6.5. Cloning
Section 6.6. Accessing Properties and Methods
Section 6.7. Static Class Members
Section 6.8. Access Types
Section 6.9. Binding
Section 6.10. Abstract Methods and Abstract Classes
Section 6.11. User-Level Overloading
Section 6.12. Class Autoloading
Section 6.13. Object Serialization
Section 6.14. Namespaces
Section 6.15. The Evolution of the Zend Engine
Chapter 7. I/O and Disk Access
Section 7.1. HTTP Connections
Section 7.2. Writing to the Browser
Section 7.3. Output Buffering
Section 7.4. Environment Variables
Section 7.5. Getting Input from Forms
Section 7.6. Passing Arrays in Forms
Section 7.7. Cookies
Section 7.8. File Uploads
Section 7.9. Reading and Writing to Files
Section 7.10. Sessions
Section 7.11. The include and require Functions
Section 7.12. Don't Trust User Input
Part II. Functional Reference
Chapter 8. Browser I/O
Section 8.1. Pregenerated Variables
Section 8.2. Pregenerated Constants
Section 8.3. Sending Text to the Browser
Section 8.4. Output Buffering
Section 8.5. Session Handling
Section 8.6. HTTP Headers
Chapter 9. Operating System
Section 9.1. Files
Section 9.2. Compressed File Functions
Section 9.3. Direct I/O
Section 9.4. Debugging
Section 9.5. POSIX
Section 9.6. Shell Commands
Section 9.7. Process Control
Chapter 10. Network I/O
Section 10.1. General Network I/O
Section 10.2. Sockets
Section 10.3. FTP
Section 10.4. Curl
Section 10.5. SNMP
Chapter 11. Data
Section 11.1. Data Types, Constants, and Variables
Section 11.2. Arrays
Section 11.3. Objects and Classes
Section 11.4. User Defined Functions
Chapter 12. Encoding and Decoding
Section 12.1. Strings
Section 12.2. String Comparison
Section 12.3. Encoding and Decoding
Section 12.4. Compression
Section 12.5. Encryption
Section 12.6. Hashing
Section 12.7. Spell Checking
Section 12.8. Regular Expressions
Section 12.9. Character Set Encoding
Chapter 13. Math
Section 13.1. Common Math
Section 13.2. Random Numbers
Section 13.3. Arbitrary-Precision Numbers
Chapter 14. Time and Date
Section 14.1. Time and Date
Section 14.2. Alternative Calendars
Chapter 15. Configuration
Section 15.1. Configuration Directives
Section 15.2. Configuration
Chapter 16. Images and Graphics
Section 16.1. Analyzing Images
Section 16.2. Creating Images
Chapter 17. Database
Section 17.1. DBM-Style Database Abstraction
Section 17.2. DBX
Section 17.3. LDAP
Section 17.4. MySQL
Section 17.5. ODBC
Section 17.6. Oracle
Section 17.7. Postgres
Section 17.8. Sybase and Microsoft SQL Server
Chapter 18. Object Layers
Section 18.1. COM
Section 18.2. CORBA
Section 18.3. Java
Chapter 19. Miscellaneous
Section 19.1. Apache
Section 19.2. IMAP
Section 19.3. MnoGoSearch
Section 19.4. OpenSSL
Section 19.5. System V Messages
Section 19.6. System V Semaphores
Section 19.7. System V Shared Memory
Chapter 20. XML
Section 20.1. DOM XML
Section 20.2. Expat XML
Section 20.3. WDDX
Part III. Algorithms
Chapter 21. Sorting, Searching, and Random Numbers
Section 21.1. Sorting
Section 21.2. Built-In Sorting Functions
Section 21.3. Sorting with a Comparison Function
Section 21.4. Searching
Section 21.5. Indexing
Section 21.6. Random Numbers
Section 21.7. Random Identifiers
Section 21.8. Choosing Banner Ads
Chapter 22. Parsing and String Evaluation
Section 22.1. Tokenizing
Section 22.2. Regular Expressions
Section 22.3. Defining Regular Expressions
Section 22.4. Using Regular Expressions in PHP Scripts
Chapter 23. Database Integration
Section 23.1. Building HTML Tables from SQL Queries
Section 23.2. Tracking Visitors with Session Identifiers
Section 23.3. Storing Content in a Database
Section 23.4. Database Abstraction Layers
Chapter 24. Networks
Section 24.1. HTTP Authentication
Section 24.2. Controlling the Browser's Cache
Section 24.3. Setting Document Type
Section 24.4. Email with Attachments
Section 24.5. HTML Email
Section 24.6. Verifying an Email Address
Chapter 25. Generating Graphics
Section 25.1. Dynamic Buttons
Section 25.2. Generating Graphs on the Fly
Section 25.3. Bar Graphs
Section 25.4. Pie Charts
Section 25.5. Stretching Single-Pixel Images
Part IV. Software Engineering
Chapter 26. Integration with HTML
Section 26.1. Sprinkling PHP within an HTML Document
Section 26.2. Using PHP to Output All HTML
Section 26.3. Separating HTML from PHP
Section 26.4. Generating HTML with PHP
Chapter 27. Design
Section 27.1. Writing Requirements Specifications
Section 27.2. Writing Design Documents
Section 27.3. Change Management
Section 27.4. Modularization Using include
Section 27.5. FreeEnergy
Section 27.6. Templates
Section 27.7. Application Frameworks
Section 27.8. PEAR
Section 27.9. URLs Friendly to Search Engines
Chapter 28. Efficiency and Debugging
Section 28.1. Optimization
Section 28.2. Measuring Performance
Section 28.3. Optimize the Slowest Parts
Section 28.4. When to Store Content in a Database
Section 28.5. Debugging Strategies
Section 28.6. Simulating HTTP Connections
Section 28.7. Output Buffering
Section 28.8. Output Compression
Section 28.9. Avoiding eval
Section 28.10. Don't Load Extensions Dynamically
Section 28.11. Improving Performance of MySQL Queries
Section 28.12. Optimizing Disk-Based Sessions
Section 28.13. Don't Pass by Reference (or, Don't Trust Your Instincts)
Section 28.14. Avoid Concatenation of Large Strings
Section 28.15. Avoid Serving Large Files with PHP-Enabled Apache
Section 28.16. Understanding Persistent Database Connections
Section 28.17. Avoid Using exec, Backticks, and system If Possible
Section 28.18. Use php.ini-recommended
Section 28.19. Don't Use Regular Expressions Unless You Must
Section 28.20. Optimizing Loops
Section 28.21. IIS Configuration
Chapter 29. Design Patterns
Section 29.1. Patterns Defined
Section 29.2. Singleton
Section 29.3. Factory
Section 29.4. Observer
Section 29.5. Strategy
Appendix A. Escape Sequences
Appendix B. ASCII Codes
Appendix C. Operators
Appendix D. PHP Tags
Appendix E. PHP Compile-Time Configuration
Appendix F. Internet Resources
Section F.1. Portals
Section F.2. Software
Appendix G. PHP Style Guide
Section G.1. Comments
Section G.2. Function Declarations
Section G.3. Compound Statements
Section G.4. Naming
Section G.5. Expressions
Index
Бесплатно скачать электронную книгу в удобном формате, смотреть и читать:
Скачать книгу Core PHP Programming, Third Edition, Atkinson L. - fileskachat.com, быстрое и бесплатное скачивание.
Скачать chm
Ниже можно купить эту книгу, если она есть в продаже, и похожие книги по лучшей цене со скидкой с доставкой по всей России.Купить книги
Скачать книгу Core PHP Programming - Third Edition - Leon Atkinson
Скачать книгу Core PHP Programming - Third Edition - Leon Atkinson - depositfiles
Дата публикации:
Теги: программирование :: PHP :: обучение PHP :: самоучитель по PHP :: Аткинсон :: Atkinson :: core PHP programming :: PHP programming :: самоучитель PHP :: обучение на примерах :: основы PHP :: PHP для начинающих :: язык программирования PHP :: программирование на PHP :: примеры программирования на PHP :: CGI-сценарии :: сервер Apache :: РНР-функции :: cookie :: сеансы :: FTP :: e-mail :: базы данных :: MySQL :: ООП :: Web-приложения :: строки :: массивы :: книга :: операторы :: скачать
Смотрите также учебники, книги и учебные материалы:
Следующие учебники и книги:
Предыдущие статьи: