PHP & MySQL Web Development teaches the reader to develop dynamic, secure e-commerce Web sites and Web applications. The book shows how to integrate and implement these technologies by following real-world examples and working sample projects. It also covers the related technologies needed to build a commercial Web site such as SSL, shopping carts, and payment systems. The CD includes a Linux distribution, MySQL, PHP4 and utilities for the projects and code listings.
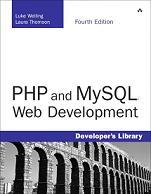
Бесплатно скачать электронную книгу в удобном формате, смотреть и читать:
Скачать книгу PHP and MySQL Web Development, Welling L., Thomson L. - fileskachat.com, быстрое и бесплатное скачивание.
Скачать файл № 1 - pdf
Скачать файл № 2 - pdf
Скачать файл № 3 - chm
Ниже можно купить эту книгу по лучшей цене со скидкой с доставкой по всей России.Купить эту книгу
Скачать книгу PHP and MySQL Web Development - Luke Welling, Laura Thomson - depositfiles
About the Authors
About the Contributors
Acknowledgments
Tell Us What You Think!
Introduction
Why You Should Read This Book
What You Will Be Able to Achieve Using This Book
What Is PHP?
What's New In PHP Version 4?
What Is MySQL?
Why Use PHP and MySQL?
Some of PHP's Strengths
Some of MySQL's Strengths
How Is This Book Organized?
1. PHP Crash Course
Using PHP
Sample Application: Bob's Auto Parts
Embedding PHP in HTML
Adding Dynamic Content
Accessing Form Variables
Identifiers
User-Declared Variables
Assigning Values to Variables
Variable Types
Constants
Variable Scope
Operators
Using Operators: Working Out the Form Totals
Precedence and Associativity: Evaluating Expressions
Variable Functions
Control Structures
Making Decisions with Conditionals
Iteration: Repeating Actions
Breaking Out of a Control Structure or Script
Next: Saving the Customer's Order
2. Storing and Retrieving Data
Saving Data for Later
Storing and Retrieving Bob's Orders
Overview of File Processing
Opening a File
Writing to a File
Closing a File
Reading from a File
Other Useful File Functions
File Locking
Doing It a Better Way: Database Management Systems
Further Reading
Next
3. Using Arrays
What Is an Array?
Numerically Indexed Arrays
Associative Arrays
Multidimensional Arrays
Sorting Arrays
Sorting Multidimensional Arrays
Reordering Arrays
Loading Arrays from Files
Other Array Manipulations
Further Reading
Next
4. String Manipulation and Regular Expressions
Example Application: Smart Form Mail
Formatting Strings
Joining and Splitting Strings with String Functions
Comparing Strings
Matching and Replacing Substrings with String Functions
Introduction to Regular Expressions
Finding Substrings with Regular Expressions
Replacing Substrings with Regular Expressions
Splitting Strings with Regular Expressions
Comparison of String Functions and Regular Expression Functions
Further Reading
Next
5. Reusing Code and Writing Functions
Why Reuse Code?
Using require() and include()
Using require() for Web Site Templates
Using Functions in PHP
Why Should You Define Your Own Functions?
Basic Function Structure
Parameters
Scope
Pass by Reference Versus Pass by Value
Returning from Functions
Returning Values from Functions
Recursion
Further Reading
Next
6. Object-Oriented PHP
Object-Oriented Concepts
Creating Classes, Attributes, Operations in PHP
Instantiation
Using Class Attributes
Calling Class Operations
Implementing Inheritance in PHP
Designing Classes
Writing the Code for Your Class
Next
7. Designing Your Web Database
Relational Database Concepts
How to Design Your Web Database
Web Database Architecture
Further Reading
Next
8. Creating Your Web Database
A Note on Using the MySQL Monitor
How to Log In to MySQL
Creating Databases and Users
Users and Privileges
Introduction to MySQL's Privilege System
Setting Up a User for the Web
Using the Right Database
Creating Database Tables
MySQL Identifiers
Column Data Types
Further Reading
Next
9. Working with Your MySQL Database
What Is SQL?
Inserting Data into the Database
Retrieving Data from the Database
Updating Records in the Database
Altering Tables After Creation
Deleting Records from the Database
Dropping Tables
Dropping a Whole Database
Further Reading
Next
10. Accessing Your MySQL Database from the Web with PHP
How Web Database Architectures Work
The Basic Steps in Querying a Database from the Web
Checking and Filtering Input Data
Setting Up a Connection
Choosing a Database to Use
Querying the Database
Retrieving the Query Results
Disconnecting from the Database
Putting New Information in the Database
Other Useful PHP-MySQL Functions
Other PHP-Database Interfaces
Further Reading
Next
11. Advanced MySQL
Understanding the Privilege System in Detail
Making Your MySQL Database Secure
Getting More Information About Databases
Speeding Up Queries with Indexes
General Optimization Tips
Different Table Types
Loading Data from a File
Further Reading
Next
12. Running an E-commerce Site
What Do You Want to Achieve?
Types of Commercial Web Sites
Risks and Threats
Deciding on a Strategy
Next
13. E-commerce Security Issues
How Important Is Your Information?
Security Threats
Balancing Usability, Performance, Cost, and Security
Creating a Security Policy
Authentication Principles
Using Authentication
Encryption Basics
Private Key Encryption
Public Key Encryption
Digital Signatures
Digital Certificates
Secure Web Servers
Auditing and Logging
Firewalls
Backing Up Data
Physical Security
Next
14. Implementing Authentication with PHP and MySQL
Identifying Visitors
Implementing Access Control
Basic Authentication
Using Basic Authentication in PHP
Using Basic Authentication with Apache's .htaccess Files
Using Basic Authentication with IIS
Using mod_auth_mysql Authentication
Creating Your Own Custom Authentication
Further Reading
Next
15. Implementing Secure Transactions with PHP and MySQL
Providing Secure Transactions
Using Secure Sockets Layer (SSL)
Screening User Input
Providing Secure Storage
Why Are You Storing Credit Card Numbers?
Using Encryption in PHP
Further Reading
Next
16. Interacting with the File System and the Server
Introduction to File Upload
Using Directory Functions
Interacting with the File System
Using Program Execution Functions
Interacting with the Environment: getenv() and putenv()
Further Reading
Next
17. Using Network and Protocol Functions
Overview of Protocols
Sending and Reading Email
Using Other Web Services
Using Network Lookup Functions
Using FTP
Generic Network Communications with cURL
Further Reading
Next
18. Managing the Date and Time
Getting the Date and Time from PHP
Converting Between PHP and MySQL Date Formats
Date Calculations
Using the Calendar Functions
Further Reading
Next
19. Generating Images
Setting Up Image Support in PHP
Image Formats
Creating Images
Using Automatically Generated Images in Other Pages
Using Text and Fonts to Create Images
Drawing Figures and Graphing Data
Other Image Functions
Further Reading
Next
20. Using Session Control in PHP
What Session Control Is
Basic Session Functionality
Implementing Simple Sessions
Simple Session Example
Configuring Session Control
Implementing Authentication with Session Control
Further Reading
Next
21. Other Useful Features
Using Magic Quotes
Evaluating Strings: eval()
Terminating Execution: die and exit
Serialization
Getting Information About the PHP Environment
Loading Extensions Dynamically
Temporarily Altering the Runtime Environment
Source Highlighting
Next
22. Using PHP and MySQL for Large Projects
Applying Software Engineering to Web Development
Planning and Running a Web Application Project
Reusing Code
Writing Maintainable Code
Implementing Version Control
Choosing a Development Environment
Documenting Your Projects
Prototyping
Separating Logic and Content
Optimizing Code
Testing
Further Reading
Next
23. Debugging
Programming Errors
Variable Debugging Aid
Error Reporting Levels
Altering the Error Reporting Settings
Triggering Your Own Errors
Handling Errors Gracefully
Remote Debugging
Next
24. Building User Authentication and Personalization
The Problem
Solution Components
Solution Overview
Implementing the Database
Implementing the Basic Site
Implementing User Authentication
Implementing Bookmark Storage and Retrieval
Implementing Recommendations
Wrapping Up and Possible Extensions
Next
25. Building a Shopping Cart
The Problem
Solution Components
Solution Overview
Implementing the Database
Implementing the Online Catalog
Implementing the Shopping Cart
Implementing Payment
Implementing an Administration Interface
Extending the Project
Using an Existing System
Next
26. Building a Content Management System
The Problem
Solution Requirements
Editing Content
Using Metadata
Formatting the Output
Image Manipulation
Solution Design/Overview
Designing the Database
Implementation
Extending the Project
Next
27. Building a Web-Based Email Service
The Problem
Solution Components
Solution Overview
Setting Up the Database
Script Architecture
Logging In and Out
Setting Up Accounts
Reading Mail
Sending Mail
Extending the Project
Next
28. Building a Mailing List Manager
The Problem
Solution Components
File Upload
Sending Mail with Attachments
Solution Overview
Setting Up the Database
Script Architecture
Implementing Login
Implementing User Functions
Implementing Administrative Functions
Extending the Project
Next
29. Building Web Forums
The Problem
Solution Components
Solution Overview
Designing the Database
Viewing the Tree of Articles
Viewing Individual Articles
Adding New Articles
Extensions
Using an Existing System
Next
30. Generating Personalized Documents in Portable Document Format (PDF)
The Problem
Evaluating Document Formats
Solution Components
Solution Overview
Problems with Headers
Extending the Project
Further Reading
A. Installing PHP 4 and MySQL
Running PHP as a CGI Interpreter or Module
Installing Apache, PHP, and MySQL Under UNIX
Installing Apache, PHP, and MySQL Under Windows
Other Configurations
B. Web Resources
PHP Resources
MySQL and SQL Specific Resources
Apache Resources
Web Development
Скачать книгу PHP and MySQL Web Development - Luke Welling, Laura Thomson
Скачать книгу PHP and MySQL Web Development - Luke Welling, Laura Thomson - depositfiles
Дата публикации:
Теги: программирование :: PHP :: обучение PHP :: самоучитель по PHP :: web development :: arrays :: Welling :: Thomson :: reusing code :: object-oriented PHP :: web database :: MySQL database :: самоучитель PHP :: обучение на примерах :: основы PHP :: PHP для начинающих :: язык программирования PHP :: программирование на PHP :: примеры программирования на PHP :: CGI-сценарии :: сервер Apache :: РНР-функции :: cookie :: сеансы :: FTP :: e-mail :: базы данных :: MySQL :: ООП :: Web-приложения :: строки :: массивы :: книга :: операторы :: скачать
Смотрите также учебники, книги и учебные материалы:
Следующие учебники и книги:
- MySQL and PHP from Scratch
- Учебник по РНР
- MySQL and JSP Web Applications, Data-Driven Programming Using Tomcat and MySQL, Turner J.
- MySQL Tutorial, Welling L., Thomson L.
Предыдущие статьи:
- PHP and PostgreSQL, Advanced Web Programming
- PHP Developer s Cookbook, Hughes S.
- Sams Teach Yourself PHP, MySQL and Apache in 24 Hours, Meloni J.C.
- Практическое руководство по SQL, Боуман Д.С., Эмерсон С.Л., Дарновски М.